AzPark: SWA - Azure Static Web Apps Authentication in Angular application
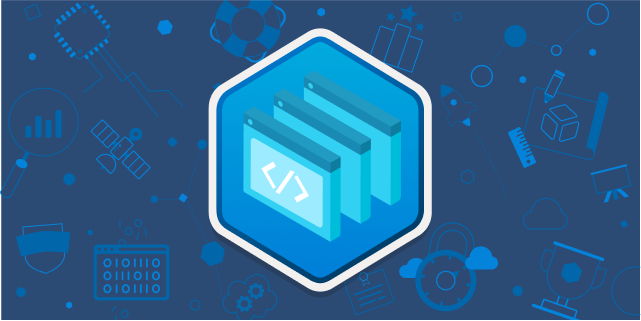
About this blog
Hey folks!, In this blog let’s see how to implement authentication flow in our Angular application and also will cover how to emulate this flow in our local development.
Azure Static Web App authentication
SWA provides a streamlined authentication experience. By default , you have access to three providers that is pre-configured or you do have option to register a custom provider
By default Azure provides us with two built-in roles
- anonymous
- authenticated
We will cover role management in a seperate blog. In this blog let’s try to focus on authentication based on default providers. Below are the default providers pre-configured
- Microsoft Active Directory
- GitHub
Login routes
Authorization provider | Login route |
---|---|
Azure Active Directory | ./auth/login/aad |
GitHub | ./auth/login/github |
./auth/login/twitter |
Authentication flow in Az-Park
Access user information
SWA provides authentication-related user information via a direct-access endpoint and to API functions. We will see how to authorize our backend in a seperate blog.
The direct-access endpoint is a utility API that exposes user information and it isn’t subject to cold start delays that are associated with serverless architecture.
Client principal data
|
|
Direct-access endpoint
We can send a GET request to the ./auth/me route that provides us with the above client principal data. For the logged-in users , the response is as same as above json , if request is from unauthenticated users then it returns null.
In your angular application expose below method in a service
|
|
Implementing CanActivate in Angular
Those who are familiar with Angular frontend development should have came across this AuthGuards . It is a concept that is used to prevent unauthenticated or unauthorized users from accessing restricted routes. It does this by implementing the CanActivate interface that allows the guard to decide if a route can be activated.
If the method returns true the request is from a authenticated user or else the route is blocked. Use below code to implement this interface.
|
|
Configure in routing (app-routing.module.ts)
|
|
Join us
Please go ahead and join our discord channel (https://discord.gg/8Cs82yNS) to give some valuable feedbacks